React Server Components in Next.js
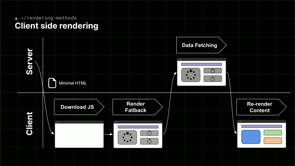
Introduction to Vercel and React Server Components
Richard Zhang, from Vercel, begins by introducing Vercel as a frontend cloud platform known for its open-source contributions, including Next.js. He highlights the close relationship between Next.js and React, particularly in the context of React Server Components, emphasizing that his talk aims to explain what React Server Components are, how they differ from existing approaches, and how to use them effectively in Next.js.
Recap of Client-Side Rendering and Server-Side Rendering
Before diving into React Server Components, Richard provides a refresher on client-side rendering (CSR) and server-side rendering (SSR). He explains that in CSR, most UI rendering happens on the client-side, leading to potential delays and SEO limitations. SSR addresses these issues by pre-rendering HTML on the server, improving initial load times and SEO. However, SSR still requires client-side hydration to add interactivity, and the entire page rendering process typically happens at the page level.
Introduction to React Server Components
Richard introduces React Server Components as a solution to some of the limitations of traditional client-side and server-side rendering. He explains that server components are rendered exclusively on the server, with their final output being React elements sent to the client. This approach eliminates the need to send server component JavaScript to the browser, reducing bundle sizes and improving performance.
Simplified Model of Server Components
Richard presents a simplified model to illustrate how server components work. In this model, data fetching occurs at the beginning, followed by HTML generation for the entire page, similar to SSR. However, the key difference is the React server component payload, which contains information about server components rendered on the server. This payload is sent to the client, where server components are directly added to the UI without requiring hydration, while client components are hydrated separately.
How Server Components Work in Next.js
Richard explains how Next.js implements server components in a more sophisticated manner compared to the simplified model. Instead of a waterfall approach, data fetching can happen within server components themselves, allowing for individual components to render as soon as their data is ready. This approach, combined with React 18 APIs like Suspense, enables progressive rendering and streaming of server components, further enhancing performance and user experience.
Benefits of Server Components
Richard summarizes the three main benefits of using server components: reduced bundle sizes due to less JavaScript being sent to the client, improved data fetching and caching capabilities by allowing components to handle their data fetching logic, and enhanced streaming capabilities through progressive rendering of server components.
Server Components vs. Client Components in Code
Richard delves into the code-level differences between server and client components. He explains that server components, by default in Next.js's App Router, can perform server-side operations like data fetching and accessing backend resources directly. Client components, on the other hand, are denoted using the 'use client' directive and are suitable for handling interactivity, browser APIs, and hooks.
Understanding Client Boundaries
Richard introduces the concept of client boundaries, explaining that when server components are imported into client components, they are automatically converted into client components. This behavior is due to the way React handles component boundaries and the need for a clear separation between server-rendered and client-rendered parts of the application.
Hoisting Server Components to Maintain Server-Side Rendering
Richard demonstrates how to prevent server components from being converted into client components when used within client components. The solution involves "hoisting" the server components by passing them as props to the client component, ensuring that React recognizes and renders them on the server. While acknowledging that this approach might seem less elegant, he emphasizes that it's a necessary step to maintain the benefits of server-side rendering for those specific components.
Where to Go From Here and Exploring Server Components in Next.js
Richard concludes by suggesting ways to explore server components further, recommending Next.js's App Router as a starting point. He highlights Vercel's commitment to iterating on its technologies based on feedback and encourages the audience to experiment with server components and provide feedback. He also advises looking into React Suspense for a deeper understanding of how Next.js integrates these concepts to enhance performance and developer experience.
Who here's heard of Vercel or has used Vercel?
Do you wanna Raise your hands.
Okay, I've noticed my Vercel colleagues here are raising their hands.
I'd be pretty worried if you didn't know about Vercel.
But yeah, for those that haven't heard about Vercel, we do a bunch of different things, but Vercel itself are a front end cloud platform, so if you want to deploy web applications very very quickly online you'd use Vercel for that.
And, we also have a really strong open source presence.
We create and maintain some of the world's most widely used open source libraries.
One of those actually being NextJS.
And NextJS has, in some ways, a really close relationship with React server components, or React in general.
And I'm glad that Julian did a talk about React server components, because I think it fits really well into what I'm going to be talking about today.
But yeah, one of the reasons why we have a close relationship with the React kind of side of things is we talk really closely with the React team.
Three of the five original core React team members now work at Vercel.
And we get really great insights into where React is going.
And so last year we actually introduced React Server Components into NextJS.
And, like Julian was saying, yeah, like I hate talks where they're like, oh, like you need to pick up this new bleeding edge technology, React Server Components is the future.
I think my, the point of today's talk is not to convince you that React Server Components is something that you need to be using.
Again, you might have very specific use cases, unique cases.
What I want everyone to walk away from today is what exactly are React Server Components?
How do they differ from the way we've actually been doing things?
And how can you actually apply them?
How can you actually use them?
And in this case, how can you use them in NextJS.
Before I kind of dive into React's server components itself, I think it's important to do a little refresher on how we've actually been doing things.
And Julian's already spoken a lot about how we've moved from the server back to the client, back to the server.
And so I've, what I've done is I've created this amazing diagram that makes no sense really.
If you've ever spun up a sort of default React application, maybe you've used Create React App, or Vite for example, this is a very kind of oversimplified diagram of how it works, but effectively this is what, in general, at a very high level, a user kind of experiences when they hit your site.
And you can see here, with client side rendering, What client side rendering in general is most of that work of rendering the UI, rendering what's actually displayed on the browser, happens on the client side.
It happens on the user's device.
In this situation, what happens is the server sends some HTML, but the HTML document's empty.
But what it does have is a reference to some JavaScript.
So this JavaScript has to actually be downloaded, because that contains all the instructions of what actually needs to be displayed to the end user.
But in most cases, if you've ever hit a site and you start seeing a ton of loading spinners, effectively, that's probably because it needs to fetch some data.
So there's some back and forth between the server and the client that needs to happen.
And then at some point, once that data's fetched, React then does its final work.
It re renders all of that data.
Sort of content.
Now, this is great in some ways because you add a lot of interactivity.
You can add interactivity.
You can use event handlers, browser APIs when you do client side rendering.
Which is what Julian was talking about.
The only downside is that for a really long time you don't actually get to see what the final outcome is.
Here you've seen an empty screen while you're downloading some JavaScript and you can imagine if your JavaScript bundle is quite large you've got a lot of dependencies, this will take some time.
The other thing is, yeah, you're seeing loading spinners, you have to make a round trip back to the server, and then finally you only get to see that content.
In many ways, not only is it a poor sort of user experience in some ways, but also you get poor SEO.
Imagine if Google was trying to index your site.
It actually has to do some work to actually find out what content is on your site.
What did teams do?
And there's many different teams that have tried to solve this issue.
And NextJS is just one of those.
And they landed on what we call server side rendering.
And, there's many different ways you can do server side rendering.
This is just one way you can structure it.
And I would even say, static site generation, what Julian was referring to, is also falls under that umbrella a little bit.
It just happens at a different time, this is happening per request, static site generation happens at build time.
But, effectively, what we're trying to eliminate is all this back and forth.
Why can't we just get the server to send some information down so that the user can actually start seeing some stuff?
Before the browser has to do the extra work to actually complete that process.
That's what server side rendering seeks to solve in this sort of problem space.
And so really the key difference here is this pre rendered HTML that you can see here.
This HTML is generated on the server so that when it's sent down, the user actually gets to see something.
And then, so then, the next step is that React kind of completes that process.
It's what we call hydration.
So then, we layer on the JavaScript, we layer on all the event handles, we layer on all of that interactivity stuff, so that the user can start interacting with the page.
This process in general is much better, because at least when the user's downloading some JavaScript, they can see something.
Whereas before, you couldn't see anything at all.
But, the thing is, you can't actually interact with it.
You still have to wait for all of that JavaScript to download, and do its step for hydration.
The other thing is in general, and, this is the way it works in NextJS before we introduced React server components, this has to happen all at a page level.
So you have to wait for each of these steps to complete.
Before you can get to this final part.
And so here you see when you're fetching data, you have to wait for all of that before you can pre render the HTML.
And fetching data, imagine if you're fetching a lot of data for the page.
This step could take quite some time.
Unless it's purely static of the page, you don't expect the page to change.
You could do static site generation, that would solve this issue.
But, in general, this might be problematic.
But, again, server side rendering solves a lot of issues that client side rendering had.
Which is moving part of that work to the server.
And, I think from an SEO perspective as well.
Google, when they're crawling your site, for example, they don't really need to do much work.
They'll know exactly what content is displayed on your page.
What did the React team decide to do?
And, they introduced what we called React Server Components to solve a lot of, those kind of edge cases that we were talking about.
And yeah, they looked at the server and was like, that's what I want.
Yeah, React Server Components solves, a very specific problem.
And as Julian was mentioning, it's, not something to replace server side rendering, it's something that you can use on top of it.
It's another toolkit that you can just use in your, toolset.
And the thing with what we've been talking about so far in React is we've actually only just been talking about client components.
So everything up until this point in time, we've just been talking about client components.
And the thing with, I, I feel like it's poor naming.
But the thing with client components is that either they're completely rendered on the browser, or they're partially rendered on the server and then partially rendered on the client.
So that's client side rendering and server side rendering.
With server components, they're only ever rendered on the server.
So the very, the easiest way I can think about it, and this took me some time to understand myself, is that when you're rendering a server component, the final output for that server component is the actual React stuff.
And then once that's sent down to the browser, React understands that the work has already been completed, and it doesn't need to do any extra work.
It just plonks it into the UI.
So they're only actually ever rendered on the server.
And I think we can start to see maybe there's some benefits with that.
Because if they're only ever rendered on the server, and by the way, they're only ever rendered once, which I can go into a bit later, none of the JavaScript associated with those server components are actually sent down.
So even with like server side rendering, for example, you still had to send all of the JavaScript down, even though you were doing part of the work on the server.
But with server components, we don't actually have to send that JavaScript.
Imagine if you're using, a library like MomentJS.
I'm more of a DayJS guy, because it's just smaller.
But MomentJS is a pretty huge library.
But if that's a dependency in a server component, it's not going to be sent down as part of the bundle.
Which is pretty neat.
And yeah, they don't re render, which is great.
They only ever render once.
Imagine you had like server components, being yellow, or orange maybe, yellow I think, sitting under these client components.
If this client component re renders, these server components, they don't re render.
And that kind of breaks a rule that I think Erin mentioned earlier, in her talk, which is like, client compo or children components always re render when a parent component re renders.
But with server components, they don't.
Unless you do some page navigation or something like that, they won't re render, which is a pretty efficient way of doing things.
I try to simplify my understanding of how server components work in my own framework.
This is how I would do it.
This is my own framework.
It's not NextJS.
So if you ever ask me to build a framework, don't, just don't.
But this is how I would do it, because it's silly.
But effectively the way this works is, this is a very similar model to the way I was talking about with server side rendering.
It's not really that different, we're just fetching some data at the beginning.
And then we're generating some HTML.
So at this point, it's still like at the page level.
We're not talking about components at this point in time.
And so then we pre render some HTML and we send that down, the user sees something, and then we hydrate it.
Okay.
It's pretty similar to the way I was talking about with server side rendering, but there's a key difference here.
And the key difference here is what we call a React server component payload.
And this payload is effectively some information about all the server components that are being rendered on the server.
So this work here where it's like generating like this payload, all those server components are rendered on the server in this very bad model and bad framework, which I would not recommend anyone to use.
And then it's sent down.
And they're just plonked in.
So these components here, these colored ones, I'm just saying they're server components.
And on the client, we don't have to actually do any extra work to hydrate them or do anything like that.
They're basically ready to go.
The only thing we need to do is hydrate the client components.
So these client components, they're there's more to the JavaScript, but at a very high level, you download just the client components JavaScript, and then you hydrate those client components.
But the server components, they just get plonked in.
Plonked in because they're ready to go.
So we can already see some, I feel like some benefits with server components.
We're using this on top of server side rendering, one.
So you already get the benefits of server side rendering.
The other thing is, you're only hydrating part of the page now, which saves you extra time.
And the other thing is, I think that was it pretty much.
Yeah, oh yeah, and you download less JavaScript.
Yeah, these are the main benefits with this simplified model.
But in NextJS, the way it actually works is, Instead of thinking of it as a sort of waterfall method, where you have to fetch data for the entire page and then you have to Then generate the HTML for the entire page.
Actually, you can fetch data in the server components themselves.
When you're actually rendering that component, as soon as that component's ready, you don't have to wait for the rest of the page to render.
You can just render this single component and then it's just sent down to the browser immediately.
And using all the kind of new React 18 APIs, like NextJS has wrapped it up really nicely so that you don't have to really think about it.
It's just inserted, so your HTML is not sent, or your React Server Components payload is not sent all in one go.
It's sent progressively, it's what we call streaming.
So behind the scenes it's using a lot about React Suspense and all those APIs to get this to work.
And I think, there's really three main benefits, and I've touched on this.
Bundle sizes.
Is one.
Just less JavaScript that you're sending down.
Data fetching and caching.
I mentioned that the data fetching can actually happen in the server components themselves.
I think not just from a user experience standpoint of having the components sort of house their data fetching, from a developer experience point of view also, you're keeping the data fetching exactly where it needs to be.
The other thing is, now you don't have to decide whether your entire page has to be static or your entire page has to be dynamic.
You can pick and choose how dynamic each component is.
Maybe you set different caching rules for each of those components.
Maybe one needs to be more fresh than the other.
So you have that sort of flexibility now with data fetching and caching.
And streaming as well.
Those server components being sent down as soon as they're ready.
I think is a huge bonus.
How does it actually work in practice?
And I do have some code, and I know it's late in the afternoon, so I don't want to overload everyone with too much code.
But there will be just a little bit, so I promise it's not too much.
Too much.
But at a very high level, what's actually the difference between client components and server components?
And so I mentioned before, client components, they only either render on the client, or they can be partially on the server and the client.
And server components, they're all on the server.
So when we think about client components, because part of it is happening on the browser, it means that we can do things around, adding interactivity, add, accessing the browser APIs.
Maybe you want to read, the search params, for example, in the URL.
These are the types of things that you can do with client components.
And, use hooks as well, which Aaron did an awesome talk on.
And server components are basically just the opposite of that.
Yeah, I think we're done here.
Yeah, this is, what we can actually use server components for.
We can fetch some data so that you can think of server components as more static in nature.
They don't really change much.
They're only rendered once.
They're immutable, effectively.
So you can fetch some data in them.
The other cool thing with server components is because they only ever run on the server, you can actually access your backend resources pretty much directly.
You can keep like secrets in them, you can keep, pretty sensitive information on there, so that you don't have to actually write an extra API layer just to handle that, because none of that code is sent down.
And lastly, I mentioned this earlier, keeping those large dependencies.
As well, in the server components, I think is a pretty neat thing.
I hope everyone can see this, but this is what a typical sort of server component would look like.
And so by default in NextJS, so if anyone's familiar, it's called AppRouter.
That's where we can start using server components.
This is what a server component would look like.
And by default, when you actually write a React component, In NextJS, it's going to be a server component, so in my opinion, and again, many people have a lot of opinions in this space, especially to do with React server components, I feel like, by writing server components by default, I don't have to actually have, the cognitive overload of thinking whether this can be a server component.
It's already by default a server component, and then only if I need to change it into a client component, do I then change it to a client component.
Everyone can have their own opinion about that.
These are, this is a server component.
And at first, it doesn't look too strange.
But then we start to see some pretty strange things.
For example, this async.
I don't think I've ever seen a React component do that before.
A side effect, you would chuck that in a use effect, right?
Pretty much.
We're accessing our database here.
And typically, if you wanted to do this, you would have to write an extra API layer to have that happen.
Right?.
And I've been talking a lot about, the better user experience in general, but also from a sort of developer experience standpoint, we can already see there's some sort of, savings in time that we're getting out of writing things pretty simplistically like this.
But, yeah, this is an example of a server component.
And this is a client component.
So the reason why this one is a client component is because I have to useStates.
Any hooks and stuff, you'd have to make that a client component.
The way to make it a client component is pretty easy.
You just add this use client directive at the top.
And so then this would make this a client component and so then that work would to be clear, by the way, this client component would still be server side rendered.
Part of the work being done on the server, and then the rest of it done on the browser.
I've talked about client components and server components pretty, individualistically.
And this is a blog post by Josh Comey.
I'd highly recommend reading it.
The reason why I included this is because I felt like it was a really nice way of explaining it.
Because when we talk about React components, we, can never really think of them, in isolation.
React components compose each other to build out our UI.
That's why we love React.
React, right?
Based on everything I've said so far, you'd probably think that this is true, okay, so these black ones are server components, these purple ones, they're client components, okay, I've got to use client directive here and here, based on what I've said, these two would be server, be client components, but, in fact, if I'm importing these… They'd actually turn out to be client components.
And this kind of took a while for me actually to wrap my head around too.
Because it's pretty confusing, isn't it?
It's what we call, oh, it's what Josh calls a client boundary.
And effectively, if I'm importing these server components into a parent client component, the traditional way, this would convert these into client components.
And for anyone with, I forget the name, idatic memory, or they can like visually remember things.
You probably remember seeing a React tree where there was like server components under client components.
So you probably think I'm lying now, but no, there's a way around this.
There's a way around this, and I'm going to show you this.
This is the last thing that I want to show everyone because I think it's pretty important.
Because it is one of those things where if you start adopting server components, I think it's one of those things where you have to start thinking about it in a wider picture.
This is why I want to talk about it.
Let's have a look at article content.
Let's just like dive into this code here.
And then what we're going to do is we're going to dive into article page next.
So we look in article content.
Let's have a look at this.
We're importing those two server components that we were seeing before.
And what's actually happening is, as I was, as I'm saying here, these two server components aren't going to be considered as server components.
And the reason why is because we have to look up a layer.
We have to go up a layer to the parent of this component.
So we're going to go up.
To article page, where article content is now sitting.
So let's imagine for a second we're the server and we're like rendering this server component and this isn't actually how it's happening but it's an easy way to understand conceptually like what, what is actually going on.
So article content, we're importing that, that's a client component.
Header, server component.
So when React is doing its thing and it's rendering the server component, it goes okay header, that's a server component, I'm going to do my work to get that and put it into the React server component payload.
And then send that down.
So that's ready to go.
I look at article content, and I go, oh, that's a client component.
I'm going to do part of the work, and then just leave the rest for later.
Even though article content has server components inside of it, React doesn't, oh, React on the server doesn't know that.
Because it's hidden.
It's hidden as imports inside this file.
How do we get around this?
And the way to get around this is to actually hoist it up.
What we need to do is we need to go back to article content and then convert it so that it receives some props.
So it receives some props, and those props would be those server components.
And so if we go up a layer again to article page, and we do that exercise again where we go through it again, we can see your header, it's a server component, great.
Article content, client component.
Yeah, I might leave that for later.
But actually, I need to It's got some props, and as part of the React server components payload, we actually send props down as well to these client components.
And so these two server components that are originally hidden inside our core content are now visible.
And so now we know that they're server components.
And so then this is how you end up with a structure like this, which is what we had before.
Now, I would agree that is not very elegant, right?
Let's be very realistic.
Okay, it is annoying, right?
Having to send those as props.
The thing is, that as Julian was saying before, this is a very sort of early piece of technology.
And it solves a very sort of specific set of problems.
I think, one of the things that I think we do well at Vercel is, we get feedback and we work on that.
We iterate on how we've been doing things and how we release these new pieces of tech.
What I want to emphasize is, it's available, and it's something that you can take advantage of, and it's just an extra tool that you can use.
From a sort of tech point of view, like if we're talking React specifically, children components would always typically re render if a parent component would re render.
But the thing is, you get this for free with server components.
If you ever wanted to memoize, like a children component, To make it not re render, you get this for free with Server Components.
If you wanted to actually make sure that Children Components didn't re render in the traditional way This is how you would do it, or you would use React or Memo, right?
But this is actually a way of doing that, you would hoist it.
It's not it's not like we've broken any rules with the way React does things.
It actually fits very nicely into that model.
But I think there is some improvements that can be made, which is, with all sort of new pieces of tech, is true.
Yeah, you get this sort of optimization out of the box for free.
I actually have 15 minutes left, so I feel like I should've added more, but like, where to from here?
There's actually quite a bit I wanted to talk about as well.
Where I'd start exploring, if anyone is curious, is just giving NextJS AppRouter a go.
I think, if it's something that you want to try out with server components, it's definitely one of the most accessible ways.
I think other React meta frameworks will eventually start adopting and implementing it themselves.
And, I'm interested to see how they implement it.
But for now, I think NextJS is really one of the more accessible ways to do it.
And I'd start looking into suspense as well, really, the reason why I think a lot of people use NextJS is because it adopts a lot of the React APIs very, nicely into NextJS, so that you don't have to have the overhead of actually having to think about how to set these things up yourself.
Yeah, that's where I'd start going, and again, yeah, thank you, it's been good.