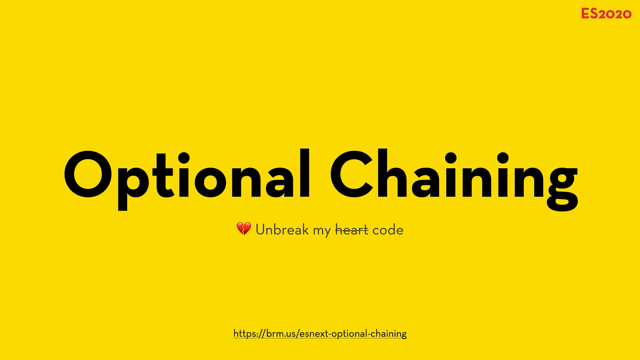
Wow, thanks for the introduction there.
It's an honor to be speaking here at this wonderful conference, and we've already seen some great stuff.
We've heard about the history of JavaScript through actually say ECMAScript.
And you've also heard about temporal a really exciting new feature that will hopefully make it into stage four anytime soon.
Now today, I won't be talking about any of the new and then spicy stuff.
I will be talking about two features that made it into ES2020, which are Optional Chaining and Null Coalescing two operators which work great hand-in-hand.
Now I want to talk about them today because they have really changed the way and how I code, and I hope they will also change the way how you code your JavaScript.
So let's take a look.
First of all the Optional Chaining Operator, what does it look like and how does it work?
Well, we'll start from the little problem that we want to solve.
So you have this object here.
It's a message.
We have a user on there in the content, and then the user have first name, location, and a Twitter handle.
Now let's select some stuff from there and we'll use console log to show it on the screen.
Now, if I select the first thing from there, of course I have to use message.user.,firstName and this will output the value that is linked into there, which is Bramus.
So that works out.
They should be known, nothing new.
Now let's select something that doesn't exist.
For example, let's select message.user.lastName.
Now the last name.
It's not there on the user object.
What will this output?
Well, this here will output undefined because the last name indeed is undefined on message.user, this is correct.
Now we can do something nice here.
We can add a fallback value if you want.
And for this, we're going to short circuit the whole thing.
So we use the double thing there the, OR operator and you say like, Hey, if the thing on the left is that something falsey?
And then we're going to fall back to the value of string with the value anonymous.
So if you run this code messenger through dot last name that evaluates to undefined, you know, that's from the previous slide.
So if you have undefined or anonymous, we get back anonymous.
Nice, I like this one.
Now let's select something inexistent-inexistent.
Here, this one gets tricky.
Let's select message.meta.publicationDate and fall back to the default value, which is the ISO string of the current date.
Now do know that message.meta that doesn't exist.
So what do we get back here?
We don't get back undefined we get back a java script error cannot read property 'publicationDate' of undefined and the undefined you see during the error, that one is actually pointing to message.meta because that's correct message.meta is not defined.
And then we want to try to read the publication date from there, but that doesn't worry because message.meta is undefined.
So the error, if that was some nonsense to you before it actually checks out, it also took me some time to like fully understand.
Now, so here we have the problem.
What is the problem?
How can we solve this?
Well, we even have a few ticks up our sleeves.
You say of course it's JavaScript, we can do some things.
For example, we could short circuit the whole thing with ampersands, and then fall back to the fallback value.
I wouldn't recommend this.
Another nasty one is where we always fall back to an empty object.
So if message doesn't exist, you fall back to an empty objects and then we try to create meta from there.
And if that doesn't exist, you fall back to an empty object.
Really, really nasty stuff.
Now, what is the proper solution?
Well, the proper solution is the Optional Chaining Operator and it looks like this.
So we have message?.meta?.publicationDate Or the new date, ISO String, . And if we invoke this line on the console, we actually get back today's date as an ISO string.
Woo Hoo, nice.
Now the Optional Chaining Operator.
Here's the definition of it.
And you have the little code example.
So, let's, let's take a look at where we can find the operator there in our code and then see how the description does it.
So we have this one here message?.
So we have it there.
And the definition says about the operator at the left-hand side of the Optional Chaining Operator evaluate undefined or null.
And then your expression evaluates undefined, or this is not the case here, because message that really does exist.
So we fall back to well targeted property access, method or function call is triggered normally.
We just continue.
The next Optional Chaining Operator that we encounter.
Again, we check the thing on the left-hand side of the operator.
What does it evaluate to?
Well, message.meta that one is undefined.
So in this case, the whole expression evaluates to undefined and the whole expression, that one being.
message.meta.publicationData that whole expression.
So that whole expression evaluates to undefined.
So we get back undefined or new data access string, and we get back at the actual date of today as an ISO string.
Cool.
Here's a little summarizing slide and there's a link there at the bottom.
Uh, no worries.
The slides will be shared online too, for you to follow.
Then a side note with this operator, it is spelled question mark period.
Not just the question mark.
So we can do crazy stuff like this.
For example, if you take a look at the last line there, we want to try to invoke a function, but we don't know if the function has been defined or not.
So this is a safe way to invoke that function.
Name of the function question mark period then between parens it's arguments.
I liked that.
Then the next thing Null Coalescing.
So we have the Optional Chaining Operator and then the Null Coalescing Operator.
That one works really well.
They work really well together.
So we have an object here, again, a message.
We have some settings on there and on the settings we have animationDuration and showSplashScreen.
So if you want to select something from there with a default value in a safe way, we know how to do this.
We use the Optional Chaining Operator for that.
So showSplashScreen, that's the one you want to select from there.
So we have messages.settings.showSplashScreen.
With optional chaining operator or fall back to true.
And what is the output of this code?
Well, unfortunately it is true, and that's not what you want because showSplashScreen.
It has a value of false.
Um, you want to have that false value from there.
Well JavaScript, of course, that's what I hate showSplashScreen.
That's false.
So we have false or true, false or true equals true, of course.
So JavaScript is technically correct here, but it's not the result that you want.
So how can we solve this?
Well, we can solve this with the introduction of the Null Coalescing Operator, which is the double question mark that we see here.
And if we execute this line on the console, we get back the actual result that you want.
Namely false.
How does this operator work?
Well, it says it's a much shorter description.
It says basically it's an equality check against nullary values, values which are null or undefined.
So we have our double question mark there, we check, the thing on the left from it is that null, or undefined?
If that is the case, then we fall back to the value on the right of it.
If that is not the case return value, which was requested.
So showSplashScreen.
It has a value of false, false ?? true That only will yield false, which is a result that we wanted to have again, summarizing slides with a little link there on the bottom.
So yeah, that was it for me for today.
I want to thank you for having me.
My name is Bramus, I also run a blog https://www.bram.us/.
It's also on Twitter.
Thanks for your attention.