Classing up ES6
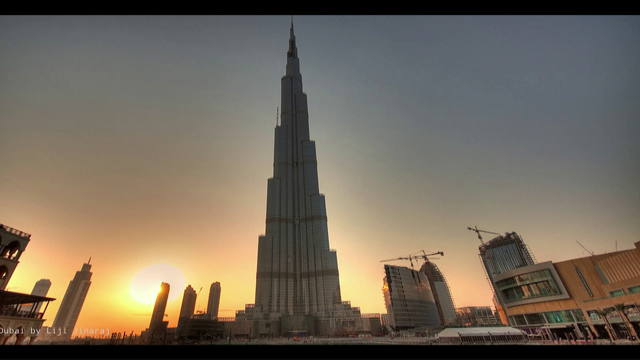
The what, why, when, where and how to of ES6 classes. What ES6 classes mean for JavaScript, but importantly the pros and cons of using them. And how you can use them today.
Let’s remember a little JS history:
- Inline scripting used to be the thing (embedded in markup).
- Then we started using the prototype.
- Then we started using JS to fix browser shortcomings (jquery).
- Then we started building on top of JS itself (client-side mvc, templating)
Through these latter stages the libraries were creating classes – but they weren’t true classes, so to speak.
ES6 Classes give…
- inheritance
- constructors
- supers
- getters/setters
- default params
- rest params (allows you to receive an unknown number of params)
- arrow functions (=>)
Isn’t all this just syntactic sugar? Yes… to an extent… but it helps create clean, understandable code. While this is possible in prototypal JS it’s easy to go wrong, or it takes a lot of time.
Support? Evergreen browsers, IE edge, node/io… but there is a lot of red in the support chart.
You end up needing transpilers, but there are transpilers (babel, traceur) so why not?
@udjamaflip
…
Jess Telford – scope chains and closures without the hand waving
1. Scope
- var – lexical scoping
- let – block scoping
- const – block scoping
1.5 Hoisting
- JS does 2 pass parsing.
- First pass (hoisting) gives scope – variables get ‘hoisted’ up to the top of the function.
- This is where lots of confusing bugs begin. Google this if you aren’t comfortable with it.
1 Scopes…
- Scopes can be nested
- You can access outer scope but not inner scope.
- You can have multiple inner scopes
- This does indeed look like a tree of scopes when you look from outer to inner (top down)
- When you look the other way, inner to outer, you get a chain (bottom up) not a tree
2 Scope chains
- If you look for a value, JS walks up the scope chain; if nothing has that value you end up in the global scope.
3 Closures
- Closures occur when an inner scope references a variable in outer scope.
- This closes over the referenced variable.
4 Garbage Collection
- GC kicks in when all the code that might need a value has been executed
5 Shadowing
- This one is a good concept but a lot of people don’t understand it too well
- When a value is defined more than once in a scope chain, JS reaches the first one and stops; the top-most scope is not closed over.
- You can avoid a lot of problems here by emulating hoisting and putting all your vars at the top of scope. Use a linter to enforce this!
If you are still not sure:
- Nodeschool.io
npm install -g scope-chains-closures
(self paced workshop)