Async and Await
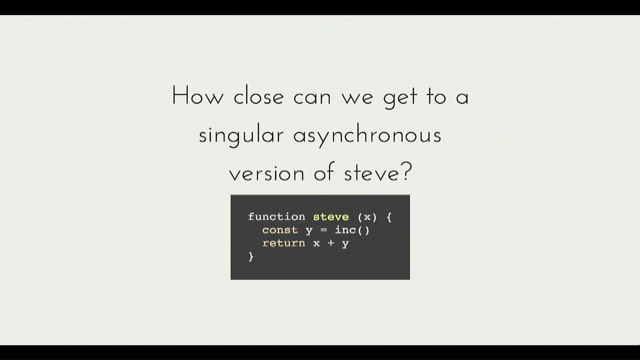
A deep exploration of proposed es2016 (AKA ES7) async functions, their inner workings (generators, promises, iterables), and how they will make our systems easier to write and maintain.
https://slides.com/jameshunter/deck-4/
Warning to functional programmers: this talk is all about side effects. Sorry!
Talking about code styles as personified friends… pretty funny 😉
But why does async have to be so hard? Why can’t we write nice simple async code?
Well you can, eg. With libraries like bluebird to set up coroutines.
But then… what is this black magic? IT’S A HACK!
Three main points….
- iterators (control the rate data is returned)
- generators
- generators again! They have an advanced mode where you can feed values back in as well as get values out
ES2016 brings in async and await to make things much clearer and easier to write (and understand).
function steve (x) { const y = inc() return x + y }
vs.
async function superSteve (x) { const y = await inc() return x + y }
(Lots of code examples, easier to look up slides!)